El TabActivity nos permite mediante Fragments tener las pestañas que queramos en el mismo Activity mostrando diferentes tipos de contenido en cada uno de ellos.
TabActivity
<?xml version="1.0" encoding="utf-8"?> <android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/main_content" android:layout_width="match_parent" android:layout_height="match_parent" android:fitsSystemWindows="true" tools:context=".TabActivity"> <android.support.design.widget.AppBarLayout android:id="@+id/appbar" android:layout_width="match_parent" android:layout_height="wrap_content" android:paddingTop="@dimen/appbar_padding_top" android:theme="@style/AppTheme.AppBarOverlay"> <android.support.v7.widget.Toolbar android:id="@+id/toolbar" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="1" app:layout_scrollFlags="scroll|enterAlways" app:popupTheme="@style/AppTheme.PopupOverlay" app:title="@string/title_activity_tabs"> </android.support.v7.widget.Toolbar> <android.support.design.widget.TabLayout android:id="@+id/tabs" android:layout_width="match_parent" android:layout_height="wrap_content"> <android.support.design.widget.TabItem android:id="@+id/tabItem" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Imagen de drawable" /> <android.support.design.widget.TabItem android:id="@+id/tabItem2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Galeria" /> </android.support.design.widget.TabLayout> </android.support.design.widget.AppBarLayout> <android.support.v4.view.ViewPager android:id="@+id/container" android:layout_width="match_parent" android:layout_height="match_parent" app:layout_behavior="@string/appbar_scrolling_view_behavior" /> </android.support.design.widget.CoordinatorLayout>
Se hace un Layout para cada Fragment con los controles que quieran y dentro del Fragment se programan las funciones que hará cada control, pero ya que los dos Fragments se muestran dentro del mismo Activity funciones como el Toast se verán igual sin importar en cual Fragment nos encontremos.
Fragment_Tab
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/constraintLayout" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <ImageView android:id="@+id/Imagen1" android:layout_width="match_parent" android:layout_height="match_parent" android:src="@drawable/youtube_boton"/> </LinearLayout>
Fragment_Tab_2
<GridLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:rowCount="3" android:columnCount="3" android:orientation="horizontal" > <ImageView android:id="@+id/Imagen2" android:layout_width="match_parent" android:layout_height="match_parent" android:src="@drawable/youtube_boton"/> </GridLayout>
En el evento onCreateView es donde creamos los Fragments
public static class PlaceholderFragment extends Fragment { /** * The fragment argument representing the section number for this * fragment. */ private static final String ARG_SECTION_NUMBER = "section_number"; public PlaceholderFragment() { } /** * Returns a new instance of this fragment for the given section * number. */ public static PlaceholderFragment newInstance(int sectionNumber) { PlaceholderFragment fragment = new PlaceholderFragment(); Bundle args = new Bundle(); args.putInt(ARG_SECTION_NUMBER, sectionNumber); fragment.setArguments(args); return fragment; } @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { View rootView = inflater.inflate(R.layout.fragment_tab, container, false); if (getArguments().getInt(ARG_SECTION_NUMBER) == 1) { rootView = inflater.inflate(R.layout.fragment_tab, container, false); } else if (getArguments().getInt(ARG_SECTION_NUMBER) == 2) { rootView = inflater.inflate(R.layout.fragment_tab2, container, false); } return rootView; } }
En la Clase SectionsPagerAdapter cargamos el Fragment correspondiente a cada sección
public class SectionsPagerAdapter extends FragmentPagerAdapter { public SectionsPagerAdapter(FragmentManager fm) { super(fm); } @Override public Fragment getItem(int position) { // getItem is called to instantiate the fragment for the given page. // Return a PlaceholderFragment (defined as a static inner class below). //return PlaceholderFragment.newInstance(position + 1); Fragment fragment = null; switch (position) { case 0: fragment = new tab(); break; case 1: fragment = new tab2(); break; } return fragment; } @Override public int getCount() { // Show 2 total pages. return 2; } }
Fragment tab
public class tab extends Fragment { ImageView Imagen; @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { // Inflate the layout for this fragment View view = inflater.inflate(R.layout.fragment_tab, container, false); Drawable myDrawable = getResources().getDrawable(R.drawable.youtube_boton); Imagen = (ImageView) view.findViewById(R.id.Imagen1); Imagen.setImageDrawable(myDrawable); return view; } }
Resultado final
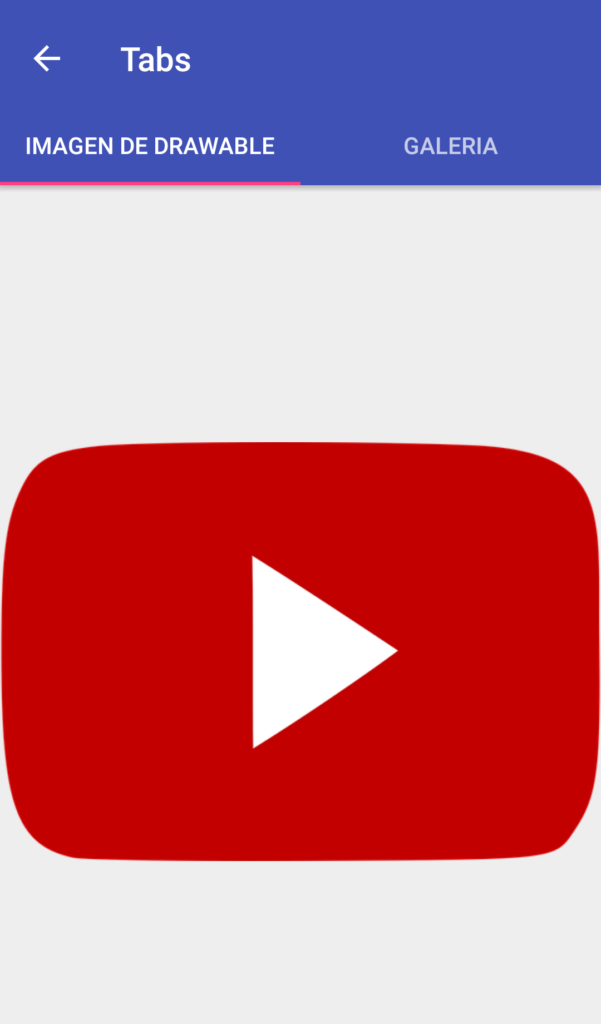